|
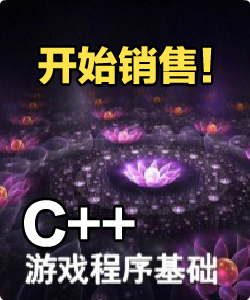 利用GUI纹理实现游戏场景切换时的渐隐效果
C#脚本- using UnityEngine;
- using System.Collections;
- public class SceneFadeInOut : MonoBehaviour
- {
- public float fadeSpeed = 1.5f; // 屏幕黑色幕布渐隐的速度.
- private bool sceneStarting = true; // 游戏场景是否开始运行.
-
- void Awake ()
- {
- // 设置纹理大小跟屏幕的大小一致
- guiTexture.pixelInset = new Rect(0f, 0f, Screen.width, Screen.height);
- }
-
-
- void Update ()
- {
- // 如果场景开始了...
- if(sceneStarting)
- // ... 就运行StartScene().
- StartScene();
- }
-
-
- void FadeToClear ()
- {
- // 这个函数主要是做:从纹理本身的颜色向透明做插值,即逐渐变淡.
- guiTexture.color = Color.Lerp(guiTexture.color, Color.clear, fadeSpeed * Time.deltaTime);
- }
-
-
- void FadeToBlack ()
- {
- //这个函数主要是做:从纹理本身的颜色向黑色做插值,即逐渐变黑.
- guiTexture.color = Color.Lerp(guiTexture.color, Color.black, fadeSpeed * Time.deltaTime);
- }
-
-
- void StartScene ()
- {
- // 执行渐隐函数.
- FadeToClear();
-
- // 判断当前的纹理颜色是不是接近透明了...
- if(guiTexture.color.a <= 0.05f)
- {
- // ...如果接近透明就直接将纹理从场景中设为不可用.
- guiTexture.color = Color.clear;
- guiTexture.enabled = false;
-
- // 设置场景开始标志位为false.
- sceneStarting = false;
- }
- }
-
-
- public void EndScene ()
- {
- // 设置纹理可用.
- guiTexture.enabled = true;
-
- // 执行从正常逐渐变黑的函数.
- FadeToBlack();
-
- // 如果场景已经足够的黑了...
- if(guiTexture.color.a >= 0.95f)
- //加载下一关的地图场景.
- Application.LoadLevel(0);
- }
- }
复制代码 JS脚本- #pragma strict
- public var fadeSpeed : float = 1.5f; // Speed that the screen fades to and from black.
- private var sceneStarting : boolean = true; // Whether or not the scene is still fading in.
- function Awake ()
- {
- // Set the texture so that it is the the size of the screen and covers it.
- guiTexture.pixelInset = new Rect(0f, 0f, Screen.width, Screen.height);
- }
- function Update ()
- {
- // If the scene is starting...
- if(sceneStarting)
- // ... call the StartScene function.
- StartScene();
- }
- function FadeToClear ()
- {
- // Lerp the colour of the texture between itself and transparent.
- guiTexture.color = Color.Lerp(guiTexture.color, Color.clear, fadeSpeed * Time.deltaTime);
- }
- function FadeToBlack ()
- {
- // Lerp the colour of the texture between itself and black.
- guiTexture.color = Color.Lerp(guiTexture.color, Color.black, fadeSpeed * Time.deltaTime);
- }
- function StartScene ()
- {
- // Fade the texture to clear.
- FadeToClear();
-
- // If the texture is almost clear...
- if(guiTexture.color.a <= 0.05f)
- {
- // ... set the colour to clear and disable the GUITexture.
- guiTexture.color = Color.clear;
- guiTexture.enabled = false;
-
- // The scene is no longer starting.
- sceneStarting = false;
- }
- }
- public function EndScene ()
- {
- // Make sure the texture is enabled.
- guiTexture.enabled = true;
-
- // Start fading towards black.
- FadeToBlack();
-
- // If the screen is almost black...
- if(guiTexture.color.a >= 0.95f)
- // ... reload the level.
- Application.LoadLevel(0);
- }
复制代码 |
|