|

楼主 |
发表于 2009-3-16 08:49:58
|
显示全部楼层
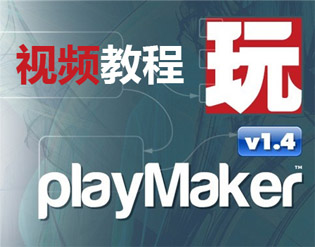 天框的使用 天框(sky box)是一种围绕观察者进行纹理映射的三维立方体的图形技术,渲染一个天框时,观察点总是定于中心位置,以便用户始终能够看到方框内部纹理映射的表面,这种技术能够制造出一种世界围绕用户的效果,如下图所示:
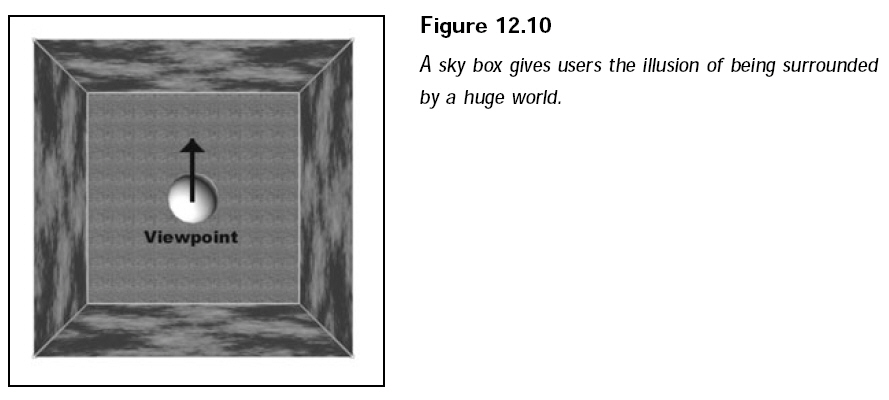 天框是非常容易是实现,只需一个立方体网格模型(其表面朝向里面)即可,通过一个顶点缓冲区存储立方体网格模型会非常不错。至于纹理,可以使用多达6个的纹理,每个表面一个纹理。网格模型并不需要很大,仅20.0单元大小的立方体就行了。纹理大小应该为256 x 256或者更高,较小的纹理将出现拉伸而且不生动。 创建一个天框类 SKY_BOX负责控制天框的每个方面,从创建渲染盒子所使用的顶点缓冲区,到渲染时所使用的纹理。 定义:
Code highlighting produced by Actipro CodeHighlighter (freeware) http://www.CodeHighlighter.com/
-->enum SKY_BOX_SIDES { TOP = 0, BOTTOM, LEFT, RIGHT, FRONT, BACK };
//===================================================================================== // This calss encapsulate how to make sky box. //===================================================================================== typedef class SKY_BOX { private: typedef struct SKY_BOX_VERTEX { float x, y, z; float u, v; } *SKY_BOX_VERTEX_PTR;
#define SKY_BOX_FVF (D3DFVF_XYZ | D3DFVF_TEX1)
private: GRAPHICS_PTR m_graphics; // parent GRAPHICS object TEXTURE m_textures[6]; // face texture (0 - 5) VERTEX_BUFFER m_vertex_buffer; // mesh vertex buffer WORLD_POSITION m_pos; // sky box position
public: SKY_BOX(); ~SKY_BOX();
BOOL create(GRAPHICS_PTR graphics); void free();
BOOL load_texture(short side, pcstr filename, D3DCOLOR transparent = 0, D3DFORMAT format = D3DFMT_UNKNOWN);
void rotate(float x_rot, float y_rot, float z_rot); void rotate_rel(float x_rot, float y_rot, float z_rot);
BOOL render(CAMERA_PTR camera, BOOL alpha_blend = FALSE); } *SKY_BOX_PTR; 实现:
Code highlighting produced by Actipro CodeHighlighter (freeware) http://www.CodeHighlighter.com/
-->//---------------------------------------------------------------------------------- // Constructor, initialize member data. //---------------------------------------------------------------------------------- SKY_BOX::SKY_BOX() { m_graphics = NULL; }
//---------------------------------------------------------------------------------- // Destructor, release allocated resource. //---------------------------------------------------------------------------------- SKY_BOX::~SKY_BOX() { free(); }
//---------------------------------------------------------------------------------- // Release allocated resource. //---------------------------------------------------------------------------------- void SKY_BOX::free() { m_graphics = NULL;
for(short i = 0; i < 6; i++) m_textures.free();
m_vertex_buffer.free(); }
//---------------------------------------------------------------------------------- // Create a sky box class object. //---------------------------------------------------------------------------------- BOOL SKY_BOX::create(GRAPHICS_PTR graphics) { SKY_BOX_VERTEX verts[24] = { { -10.0f, 10.0f, -10.0f, 0.0f, 0.0f }, // Top { 10.0f, 10.0f, -10.0f, 1.0f, 0.0f }, { -10.0f, 10.0f, 10.0f, 0.0f, 1.0f }, { 10.0f, 10.0f, 10.0f, 1.0f, 1.0f },
{ -10.0f, -10.0f, 10.0f, 0.0f, 0.0f }, // Bottom { 10.0f, -10.0f, 10.0f, 1.0f, 0.0f }, { -10.0f, -10.0f, -10.0f, 0.0f, 1.0f }, { 10.0f, -10.0f, -10.0f, 1.0f, 1.0f },
{ -10.0f, 10.0f, -10.0f, 0.0f, 0.0f }, // Left { -10.0f, 10.0f, 10.0f, 1.0f, 0.0f }, { -10.0f, -10.0f, -10.0f, 0.0f, 1.0f }, { -10.0f, -10.0f, 10.0f, 1.0f, 1.0f },
{ 10.0f, 10.0f, 10.0f, 0.0f, 0.0f }, // Right { 10.0f, 10.0f, -10.0f, 1.0f, 0.0f }, { 10.0f, -10.0f, 10.0f, 0.0f, 1.0f }, { 10.0f, -10.0f, -10.0f, 1.0f, 1.0f },
{ -10.0f, 10.0f, 10.0f, 0.0f, 0.0f }, // Front { 10.0f, 10.0f, 10.0f, 1.0f, 0.0f }, { -10.0f, -10.0f, 10.0f, 0.0f, 1.0f }, { 10.0f, -10.0f, 10.0f, 1.0f, 1.0f }, { 10.0f, 10.0f, -10.0f, 0.0f, 0.0f }, // Back { -10.0f, 10.0f, -10.0f, 1.0f, 0.0f }, { 10.0f, -10.0f, -10.0f, 0.0f, 1.0f }, { -10.0f, -10.0f, -10.0f, 1.0f, 1.0f }, };
free(); // free a prior sky box
// error checking if((m_graphics = graphics) == NULL) return FALSE;
// create the vertex buffer (and copy over sky box vertices) if(m_vertex_buffer.create(m_graphics, 24, sizeof(SKY_BOX_VERTEX), SKY_BOX_FVF)) m_vertex_buffer.set(0, 24, (void*)verts);
// rotate the sky box into default orientation rotate(0.0f, 0.0f, 0.0f);
return TRUE; }
|
|