|
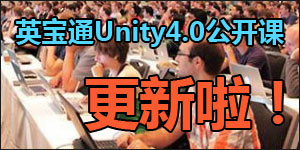 如何在游戏中创建警报灯,并通过脚本控制灯光的衰减。
--------------------------------------------------------------------------------------------------------------------------
书本原文节选:
--------------------------------------------------------------------------------------------------------------------------
C#代码- using UnityEngine;
- using System.Collections;
- public class AlarmLight : MonoBehaviour
- {
- public float fadeSpeed = 2f; // 光渐渐淡化的速度.
- public float highIntensity = 2f; // 报警灯最高的亮度.
- public float lowIntensity = 0.5f; // 报警灯最低的亮度.
- public float changeMargin = 0.2f; // 目标强度变化的范围.
- public bool alarmOn; // 报警灯是否被激活.
- private float targetIntensity; // 当前光强度
-
-
- void Awake ()
- {
- // 当游戏刚刚启动的时候,报警灯的强度为0,将不会对场景产生影响
- light.intensity = 0f;
-
- // 当第一次警报灯被激活的时候, 警报色应该是最亮的
- targetIntensity = highIntensity;
- }
-
-
- void Update ()
- {
- // 当警报灯激活响起
- if(alarmOn)
- {
- // ... 循环操作光的从当前强度变为targetIntensity强度
- light.intensity = Mathf.Lerp(light.intensity, targetIntensity, fadeSpeed * Time.deltaTime);
-
- // 切换光强变弱还是从弱变强
- CheckTargetIntensity();
- }
- else
- // 如果警报没有激活则将警报色强度渐变为0.
- light.intensity = Mathf.Lerp(light.intensity, 0f, fadeSpeed * Time.deltaTime);
- }
-
-
- void CheckTargetIntensity ()
- {
- // 如果灯光当前的亮度接近亮度范围的一端了,则需要将当targetIntensity颜色变成另外一个端的颜色....
- if(Mathf.Abs(targetIntensity - light.intensity) < changeMargin)
- {
- // ... 如果当前的灯光已经最亮了...
- if(targetIntensity == highIntensity)
- // ... 则将targetIntensity的值变成最低.
- targetIntensity = lowIntensity;
- else
- //否则将targetIntensity的值变成最高.
- targetIntensity = highIntensity;
- }
- }
- }
复制代码 JS代码- #pragma strict
- public var fadeSpeed : float = 2f; // How fast the light fades between intensities.
- public var highIntensity : float = 2f; // The maximum intensity of the light whilst the alarm is on.
- public var lowIntensity : float = 0.5f; // The minimum intensity of the light whilst the alarm is on.
- public var changeMargin : float = 0.2f; // The margin within which the target intensity is changed.
- public var alarmOn : boolean; // Whether or not the alarm is on.
- private var targetIntensity : float; // The intensity that the light is aiming for currently.
- function Awake ()
- {
- // When the level starts we want the light to be "off".
- light.intensity = 0f;
-
- // When the alarm starts for the first time, the light should aim to have the maximum intensity.
- targetIntensity = highIntensity;
- }
- function Update ()
- {
- // If the light is on...
- if(alarmOn)
- {
- // ... Lerp the light's intensity towards the current target.
- light.intensity = Mathf.Lerp(light.intensity, targetIntensity, fadeSpeed * Time.deltaTime);
-
- // Check whether the target intensity needs changing and change it if so.
- CheckTargetIntensity();
- }
- else
- // Otherwise fade the light's intensity to zero.
- light.intensity = Mathf.Lerp(light.intensity, 0f, fadeSpeed * Time.deltaTime);
- }
- function CheckTargetIntensity ()
- {
- // If the difference between the target and current intensities is less than the change margin...
- if(Mathf.Abs(targetIntensity - light.intensity) < changeMargin)
- {
- // ... if the target intensity is high...
- if(targetIntensity == highIntensity)
- // ... then set the target to low.
- targetIntensity = lowIntensity;
- else
- // Otherwise set the targer to high.
- targetIntensity = highIntensity;
- }
- }
复制代码 涉及到的知识点:
灯光 Light
|
|